# Copyright 2021 NVIDIA Corporation. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
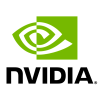
Movie Poster Feature Extraction with ResNet
In this notebook, we will use a pretrained ResNet-50 network to extract image features from the movie poster images.
Note: this notebook should be executed from within the nvidia_resnet50
container, built as follows
git clone https://github.com/NVIDIA/DeepLearningExamples
git checkout 5d6d417ff57e8824ef51573e00e5e21307b39697
cd DeepLearningExamples/PyTorch/Classification/ConvNets
docker build . -t nvidia_resnet50
Start the container, mounting the current directory:
nvidia-docker run --rm --net=host -it -v $PWD:/workspace --ipc=host nvidia_resnet50
Then from within the container:
cd /workspace
jupyter-lab --allow-root --ip='0.0.0.0'
Download a pretrained ResNet-50 from NVIDIA GPU cloud
First, we install an extra package and restart the kernel.
!pip install ipywidgets tqdm
import IPython
IPython.Application.instance().kernel.do_shutdown(True)
from PIL import Image
import argparse
import numpy as np
import json
import torch
from torch.cuda.amp import autocast
import torch.backends.cudnn as cudnn
import sys
sys.path.append('/workspace/DeepLearningExamples/PyTorch/Classification/ConvNets')
from image_classification import models
import torchvision.transforms as transforms
from image_classification.models import (
resnet50,
resnext101_32x4d,
se_resnext101_32x4d,
efficientnet_b0,
efficientnet_b4,
efficientnet_widese_b0,
efficientnet_widese_b4,
efficientnet_quant_b0,
efficientnet_quant_b4,
)
def available_models():
models = {
m.name: m
for m in [
resnet50,
resnext101_32x4d,
se_resnext101_32x4d,
efficientnet_b0,
efficientnet_b4,
efficientnet_widese_b0,
efficientnet_widese_b4,
efficientnet_quant_b0,
efficientnet_quant_b4,
]
}
return models
def load_jpeg_from_file(path, image_size, cuda=True):
img_transforms = transforms.Compose(
[
transforms.Resize(image_size + 32),
transforms.CenterCrop(image_size),
transforms.ToTensor(),
]
)
img = img_transforms(Image.open(path))
with torch.no_grad():
# mean and std are not multiplied by 255 as they are in training script
# torch dataloader reads data into bytes whereas loading directly
# through PIL creates a tensor with floats in [0,1] range
mean = torch.tensor([0.485, 0.456, 0.406]).view(1, 3, 1, 1)
std = torch.tensor([0.229, 0.224, 0.225]).view(1, 3, 1, 1)
if cuda:
mean = mean.cuda()
std = std.cuda()
img = img.cuda()
img = img.float()
if img.shape[0] == 1: #mono image
#pad channels
img = img.repeat([3, 1, 1])
input = img.unsqueeze(0).sub_(mean).div_(std)
return input
def check_quant_weight_correctness(checkpoint_path, model):
state_dict = torch.load(checkpoint_path, map_location=torch.device('cpu'))
state_dict = {k[len("module."):] if k.startswith("module.") else k: v for k, v in state_dict.items()}
quantizers_sd_keys = {f'{n[0]}._amax' for n in model.named_modules() if 'quantizer' in n[0]}
sd_all_keys = quantizers_sd_keys | set(model.state_dict().keys())
assert set(state_dict.keys()) == sd_all_keys, (f'Passed quantized architecture, but following keys are missing in '
f'checkpoint: {list(sd_all_keys - set(state_dict.keys()))}')
imgnet_classes = np.array(json.load(open("/workspace/DeepLearningExamples/PyTorch/Classification/ConvNets/LOC_synset_mapping.json", "r")))
model_args = {}
model_args["pretrained_from_file"] = './nvidia_resnet50_200821.pth.tar'
model = available_models()['resnet50'](model_args)
model = model.cuda()
model.eval()
Downloading: "https://api.ngc.nvidia.com/v2/models/nvidia/resnet50_pyt_amp/versions/20.06.0/files/nvidia_resnet50_200821.pth.tar" to /root/.cache/torch/hub/checkpoints/nvidia_resnet50_200821.pth.tar
ResNet(
(conv1): Conv2d(3, 64, kernel_size=(7, 7), stride=(2, 2), padding=(3, 3), bias=False)
(bn1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
(maxpool): MaxPool2d(kernel_size=3, stride=2, padding=1, dilation=1, ceil_mode=False)
(layer1): Sequential(
(0): Bottleneck(
(conv1): Conv2d(64, 64, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(64, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
(downsample): Sequential(
(0): Conv2d(64, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(1): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
)
)
(1): Bottleneck(
(conv1): Conv2d(256, 64, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(64, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(2): Bottleneck(
(conv1): Conv2d(256, 64, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(64, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(64, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
)
(layer2): Sequential(
(0): Bottleneck(
(conv1): Conv2d(256, 128, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(128, 128, kernel_size=(3, 3), stride=(2, 2), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(128, 512, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
(downsample): Sequential(
(0): Conv2d(256, 512, kernel_size=(1, 1), stride=(2, 2), bias=False)
(1): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
)
)
(1): Bottleneck(
(conv1): Conv2d(512, 128, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(128, 128, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(128, 512, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(2): Bottleneck(
(conv1): Conv2d(512, 128, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(128, 128, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(128, 512, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(3): Bottleneck(
(conv1): Conv2d(512, 128, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(128, 128, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(128, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(128, 512, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
)
(layer3): Sequential(
(0): Bottleneck(
(conv1): Conv2d(512, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(256, 256, kernel_size=(3, 3), stride=(2, 2), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(256, 1024, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(1024, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
(downsample): Sequential(
(0): Conv2d(512, 1024, kernel_size=(1, 1), stride=(2, 2), bias=False)
(1): BatchNorm2d(1024, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
)
)
(1): Bottleneck(
(conv1): Conv2d(1024, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(256, 256, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(256, 1024, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(1024, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(2): Bottleneck(
(conv1): Conv2d(1024, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(256, 256, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(256, 1024, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(1024, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(3): Bottleneck(
(conv1): Conv2d(1024, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(256, 256, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(256, 1024, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(1024, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(4): Bottleneck(
(conv1): Conv2d(1024, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(256, 256, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(256, 1024, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(1024, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(5): Bottleneck(
(conv1): Conv2d(1024, 256, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(256, 256, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(256, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(256, 1024, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(1024, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
)
(layer4): Sequential(
(0): Bottleneck(
(conv1): Conv2d(1024, 512, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(512, 512, kernel_size=(3, 3), stride=(2, 2), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(512, 2048, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(2048, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
(downsample): Sequential(
(0): Conv2d(1024, 2048, kernel_size=(1, 1), stride=(2, 2), bias=False)
(1): BatchNorm2d(2048, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
)
)
(1): Bottleneck(
(conv1): Conv2d(2048, 512, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(512, 512, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(512, 2048, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(2048, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
(2): Bottleneck(
(conv1): Conv2d(2048, 512, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn1): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv2): Conv2d(512, 512, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1), bias=False)
(bn2): BatchNorm2d(512, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(conv3): Conv2d(512, 2048, kernel_size=(1, 1), stride=(1, 1), bias=False)
(bn3): BatchNorm2d(2048, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
(relu): ReLU(inplace=True)
)
)
(avgpool): AdaptiveAvgPool2d(output_size=1)
(fc): Linear(in_features=2048, out_features=1000, bias=True)
)
Extract features for all movies
Next, we will extract feature for all movie posters, using the last layer just before the classification head, containing 2048 float values.
import glob
filelist = glob.glob('./poster_small/*.jpg')
len(filelist)
61951
filelist[:10]
['./poster_small/0055323.jpg',
'./poster_small/0114709.jpg',
'./poster_small/0274711.jpg',
'./poster_small/0055320.jpg',
'./poster_small/0054197.jpg',
'./poster_small/1791658.jpg',
'./poster_small/1288589.jpg',
'./poster_small/0365653.jpg',
'./poster_small/2324928.jpg',
'./poster_small/6000478.jpg']
from tqdm import tqdm
batchsize = 64
num_bathces = len(filelist)//batchsize
batches = np.array_split(filelist, num_bathces)
### strip the last layer
feature_extractor = torch.nn.Sequential(*list(model.children())[:-1])
feature_dict = {}
error = 0
for batch in tqdm(batches):
inputs = []
imgs = []
for i, f in enumerate(batch):
try:
img = load_jpeg_from_file(f, 224, cuda=True)
imgs.append(f.split('/')[-1].split('.')[0])
inputs.append(img.squeeze())
except Exception as e:
print(e)
error +=1
features = feature_extractor(torch.stack(inputs, dim=0)).cpu().detach().numpy().squeeze()
for i, f in enumerate(imgs):
feature_dict[f] =features[i,:]
print('Unable to extract features for %d images'%error)
0%| | 0/967 [00:00<?, ?it/s]
cannot identify image file './poster_small/0114709.jpg'
cannot identify image file './poster_small/0274711.jpg'
cannot identify image file './poster_small/0055320.jpg'
cannot identify image file './poster_small/0054197.jpg'
cannot identify image file './poster_small/1791658.jpg'
cannot identify image file './poster_small/1288589.jpg'
cannot identify image file './poster_small/0365653.jpg'
cannot identify image file './poster_small/2324928.jpg'
cannot identify image file './poster_small/6000478.jpg'
cannot identify image file './poster_small/0168199.jpg'
cannot identify image file './poster_small/0118926.jpg'
cannot identify image file './poster_small/0415856.jpg'
0%| | 2/967 [00:02<21:58, 1.37s/it]
cannot identify image file './poster_small/0494260.jpg'
0%| | 3/967 [00:03<18:50, 1.17s/it]
cannot identify image file './poster_small/0810772.jpg'
0%| | 4/967 [00:03<15:46, 1.02it/s]
cannot identify image file './poster_small/0049314.jpg'
1%| | 8/967 [00:06<12:59, 1.23it/s]
cannot identify image file './poster_small/0066831.jpg'
1%| | 9/967 [00:07<12:22, 1.29it/s]
cannot identify image file './poster_small/0888693.jpg'
1%| | 12/967 [00:10<14:20, 1.11it/s]
cannot identify image file './poster_small/0067431.jpg'
1%|▏ | 14/967 [00:11<13:07, 1.21it/s]
cannot identify image file './poster_small/6522546.jpg'
cannot identify image file './poster_small/0057811.jpg'
2%|▏ | 16/967 [00:13<13:05, 1.21it/s]
cannot identify image file './poster_small/5176252.jpg'
cannot identify image file './poster_small/0112373.jpg'
2%|▏ | 18/967 [00:14<10:45, 1.47it/s]
cannot identify image file './poster_small/4636254.jpg'
2%|▏ | 19/967 [00:15<11:11, 1.41it/s]
cannot identify image file './poster_small/0365658.jpg'
2%|▏ | 24/967 [00:19<13:04, 1.20it/s]
cannot identify image file './poster_small/2124046.jpg'
4%|▎ | 34/967 [00:26<12:05, 1.29it/s]
cannot identify image file './poster_small/0104469.jpg'
4%|▍ | 37/967 [00:29<13:35, 1.14it/s]
cannot identify image file './poster_small/0102493.jpg'
4%|▍ | 41/967 [00:33<13:08, 1.17it/s]
cannot identify image file './poster_small/0051792.jpg'
5%|▍ | 46/967 [00:36<09:19, 1.65it/s]
cannot identify image file './poster_small/0110017.jpg'
cannot identify image file './poster_small/0139630.jpg'
5%|▍ | 48/967 [00:37<08:21, 1.83it/s]
cannot identify image file './poster_small/0143348.jpg'
5%|▌ | 51/967 [00:38<07:56, 1.92it/s]
cannot identify image file './poster_small/0037618.jpg'
cannot identify image file './poster_small/0040002.jpg'
5%|▌ | 53/967 [00:39<08:56, 1.70it/s]
cannot identify image file './poster_small/0317950.jpg'
6%|▌ | 54/967 [00:40<10:00, 1.52it/s]
cannot identify image file './poster_small/0850669.jpg'
cannot identify image file './poster_small/0325258.jpg'
6%|▌ | 58/967 [00:44<12:21, 1.23it/s]
cannot identify image file './poster_small/6569888.jpg'
cannot identify image file './poster_small/0037736.jpg'
6%|▋ | 61/967 [00:46<12:33, 1.20it/s]
cannot identify image file './poster_small/0109303.jpg'
7%|▋ | 66/967 [00:50<10:24, 1.44it/s]
cannot identify image file './poster_small/0103882.jpg'
7%|▋ | 67/967 [00:50<09:26, 1.59it/s]
cannot identify image file './poster_small/0267287.jpg'
7%|▋ | 71/967 [00:53<12:02, 1.24it/s]
cannot identify image file './poster_small/0100033.jpg'
8%|▊ | 77/967 [00:57<10:21, 1.43it/s]
cannot identify image file './poster_small/1601215.jpg'
8%|▊ | 81/967 [01:00<10:08, 1.46it/s]
cannot identify image file './poster_small/0092028.jpg'
cannot identify image file './poster_small/0075963.jpg'
cannot identify image file './poster_small/3267334.jpg'
9%|▊ | 84/967 [01:02<08:43, 1.69it/s]
cannot identify image file './poster_small/0059398.jpg'
9%|▉ | 86/967 [01:03<08:15, 1.78it/s]
cannot identify image file './poster_small/0122565.jpg'
10%|█ | 97/967 [01:09<07:56, 1.82it/s]
cannot identify image file './poster_small/0052572.jpg'
11%|█ | 102/967 [01:12<10:25, 1.38it/s]
cannot identify image file './poster_small/6404896.jpg'
11%|█ | 103/967 [01:13<11:14, 1.28it/s]
cannot identify image file './poster_small/0027428.jpg'
cannot identify image file './poster_small/0033883.jpg'
11%|█ | 104/967 [01:14<10:13, 1.41it/s]
cannot identify image file './poster_small/0113270.jpg'
11%|█ | 108/967 [01:18<12:33, 1.14it/s]
cannot identify image file './poster_small/0022286.jpg'
12%|█▏ | 112/967 [01:21<11:23, 1.25it/s]
cannot identify image file './poster_small/0068953.jpg'
12%|█▏ | 114/967 [01:23<12:35, 1.13it/s]
cannot identify image file './poster_small/0042949.jpg'
cannot identify image file './poster_small/0130297.jpg'
12%|█▏ | 115/967 [01:24<12:22, 1.15it/s]
cannot identify image file './poster_small/0028207.jpg'
12%|█▏ | 117/967 [01:26<12:46, 1.11it/s]
cannot identify image file './poster_small/0054244.jpg'
12%|█▏ | 118/967 [01:27<12:54, 1.10it/s]
cannot identify image file './poster_small/1275680.jpg'
12%|█▏ | 120/967 [01:30<18:32, 1.31s/it]
cannot identify image file './poster_small/0036533.jpg'
cannot identify image file './poster_small/0037297.jpg'
13%|█▎ | 130/967 [01:37<09:14, 1.51it/s]
cannot identify image file './poster_small/0962736.jpg'
cannot identify image file './poster_small/0042548.jpg'
14%|█▍ | 134/967 [01:40<10:19, 1.34it/s]
cannot identify image file './poster_small/0038109.jpg'
cannot identify image file './poster_small/0104009.jpg'
14%|█▍ | 136/967 [01:41<11:21, 1.22it/s]
cannot identify image file './poster_small/0180316.jpg'
14%|█▍ | 137/967 [01:42<11:00, 1.26it/s]
cannot identify image file './poster_small/0071925.jpg'
14%|█▍ | 139/967 [01:43<10:33, 1.31it/s]
cannot identify image file './poster_small/0087001.jpg'
15%|█▍ | 143/967 [01:47<11:02, 1.24it/s]
cannot identify image file './poster_small/0056910.jpg'
15%|█▍ | 144/967 [01:47<09:55, 1.38it/s]
cannot identify image file './poster_small/0064563.jpg'
15%|█▌ | 147/967 [01:49<09:19, 1.46it/s]
cannot identify image file './poster_small/1720040.jpg'
15%|█▌ | 149/967 [01:51<12:05, 1.13it/s]
cannot identify image file './poster_small/0041112.jpg'
16%|█▌ | 156/967 [01:58<11:37, 1.16it/s]
cannot identify image file './poster_small/4412528.jpg'
16%|█▌ | 157/967 [01:58<10:57, 1.23it/s]
cannot identify image file './poster_small/0051362.jpg'
16%|█▋ | 158/967 [01:59<10:15, 1.31it/s]
cannot identify image file './poster_small/0029992.jpg'
17%|█▋ | 160/967 [02:00<08:26, 1.59it/s]
cannot identify image file './poster_small/0384309.jpg'
cannot identify image file './poster_small/0028367.jpg'
17%|█▋ | 162/967 [02:01<08:56, 1.50it/s]
cannot identify image file './poster_small/0038336.jpg'
17%|█▋ | 163/967 [02:02<10:07, 1.32it/s]
cannot identify image file './poster_small/0058725.jpg'
17%|█▋ | 164/967 [02:04<11:26, 1.17it/s]
cannot identify image file './poster_small/0113328.jpg'
17%|█▋ | 166/967 [02:05<09:21, 1.43it/s]
cannot identify image file './poster_small/3878542.jpg'
17%|█▋ | 167/967 [02:06<10:00, 1.33it/s]
cannot identify image file './poster_small/0026465.jpg'
17%|█▋ | 169/967 [02:07<10:07, 1.31it/s]
cannot identify image file './poster_small/0040588.jpg'
18%|█▊ | 175/967 [02:12<10:37, 1.24it/s]
cannot identify image file './poster_small/0086984.jpg'
18%|█▊ | 178/967 [02:14<09:23, 1.40it/s]
cannot identify image file './poster_small/0309047.jpg'
19%|█▊ | 181/967 [02:16<10:52, 1.21it/s]
cannot identify image file './poster_small/0031405.jpg'
19%|█▉ | 185/967 [02:20<11:42, 1.11it/s]
cannot identify image file './poster_small/0097493.jpg'
19%|█▉ | 186/967 [02:21<11:54, 1.09it/s]
cannot identify image file './poster_small/0346336.jpg'
cannot identify image file './poster_small/0078841.jpg'
cannot identify image file './poster_small/0018795.jpg'
cannot identify image file './poster_small/9151704.jpg'
20%|█▉ | 191/967 [02:25<10:40, 1.21it/s]
cannot identify image file './poster_small/1417097.jpg'
20%|██ | 195/967 [02:29<11:12, 1.15it/s]
cannot identify image file './poster_small/0054223.jpg'
20%|██ | 196/967 [02:30<11:16, 1.14it/s]
cannot identify image file './poster_small/0117477.jpg'
21%|██ | 199/967 [02:31<07:48, 1.64it/s]
cannot identify image file './poster_small/0000041.jpg'
21%|██ | 201/967 [02:33<09:47, 1.30it/s]
cannot identify image file './poster_small/0028907.jpg'
21%|██▏ | 207/967 [02:38<10:09, 1.25it/s]
cannot identify image file './poster_small/0366179.jpg'
22%|██▏ | 209/967 [02:40<11:12, 1.13it/s]
cannot identify image file './poster_small/0109761.jpg'
22%|██▏ | 217/967 [02:45<08:58, 1.39it/s]
cannot identify image file './poster_small/7167686.jpg'
23%|██▎ | 219/967 [02:47<08:51, 1.41it/s]
cannot identify image file './poster_small/0048973.jpg'
23%|██▎ | 226/967 [02:53<10:37, 1.16it/s]
cannot identify image file './poster_small/0100112.jpg'
cannot identify image file './poster_small/3606394.jpg'
23%|██▎ | 227/967 [02:54<10:40, 1.16it/s]
cannot identify image file './poster_small/0021890.jpg'
24%|██▎ | 228/967 [02:54<10:32, 1.17it/s]
cannot identify image file './poster_small/0033874.jpg'
cannot identify image file './poster_small/0035019.jpg'
24%|██▍ | 232/967 [02:57<09:59, 1.23it/s]
cannot identify image file './poster_small/1228953.jpg'
25%|██▍ | 237/967 [03:02<09:53, 1.23it/s]
cannot identify image file './poster_small/7688990.jpg'
cannot identify image file './poster_small/0052954.jpg'
cannot identify image file './poster_small/0092159.jpg'
25%|██▌ | 242/967 [03:06<09:58, 1.21it/s]
cannot identify image file './poster_small/0094349.jpg'
cannot identify image file './poster_small/0065136.jpg'
25%|██▌ | 246/967 [03:09<09:09, 1.31it/s]
cannot identify image file './poster_small/0027805.jpg'
cannot identify image file './poster_small/0034904.jpg'
26%|██▌ | 248/967 [03:10<10:07, 1.18it/s]
cannot identify image file './poster_small/0037522.jpg'
26%|██▌ | 250/967 [03:13<11:19, 1.06it/s]
cannot identify image file './poster_small/0036301.jpg'
26%|██▋ | 254/967 [03:16<10:26, 1.14it/s]
cannot identify image file './poster_small/0037324.jpg'
26%|██▋ | 256/967 [03:17<08:46, 1.35it/s]
cannot identify image file './poster_small/0053622.jpg'
27%|██▋ | 265/967 [03:23<08:14, 1.42it/s]
cannot identify image file './poster_small/7278178.jpg'
28%|██▊ | 266/967 [03:24<08:31, 1.37it/s]
cannot identify image file './poster_small/0418239.jpg'
cannot identify image file './poster_small/0040489.jpg'
cannot identify image file './poster_small/0069280.jpg'
28%|██▊ | 269/967 [03:27<10:44, 1.08it/s]
cannot identify image file './poster_small/0049143.jpg'
29%|██▊ | 276/967 [03:33<08:21, 1.38it/s]
cannot identify image file './poster_small/0064840.jpg'
29%|██▉ | 285/967 [03:41<10:27, 1.09it/s]
cannot identify image file './poster_small/0070723.jpg'
30%|██▉ | 287/967 [03:43<09:32, 1.19it/s]
cannot identify image file './poster_small/0057997.jpg'
30%|██▉ | 289/967 [03:44<08:37, 1.31it/s]
cannot identify image file './poster_small/0056072.jpg'
31%|███ | 295/967 [03:50<10:14, 1.09it/s]
cannot identify image file './poster_small/7446332.jpg'
31%|███ | 297/967 [03:51<09:45, 1.14it/s]
cannot identify image file './poster_small/0076618.jpg'
31%|███ | 300/967 [03:55<10:41, 1.04it/s]
cannot identify image file './poster_small/0290014.jpg'
31%|███ | 302/967 [03:56<08:05, 1.37it/s]
cannot identify image file './poster_small/0347330.jpg'
31%|███▏ | 303/967 [03:56<08:07, 1.36it/s]
cannot identify image file './poster_small/0159620.jpg'
31%|███▏ | 304/967 [03:57<08:53, 1.24it/s]
cannot identify image file './poster_small/0044667.jpg'
32%|███▏ | 307/967 [04:00<10:17, 1.07it/s]
cannot identify image file './poster_small/0040190.jpg'
cannot identify image file './poster_small/3088364.jpg'
cannot identify image file './poster_small/0230367.jpg'
32%|███▏ | 309/967 [04:02<10:05, 1.09it/s]
cannot identify image file './poster_small/0037147.jpg'
32%|███▏ | 310/967 [04:03<09:15, 1.18it/s]
cannot identify image file './poster_small/0033282.jpg'
cannot identify image file './poster_small/4028134.jpg'
32%|███▏ | 312/967 [04:05<10:09, 1.07it/s]
cannot identify image file './poster_small/1352824.jpg'
32%|███▏ | 314/967 [04:06<08:53, 1.22it/s]
cannot identify image file './poster_small/0079400.jpg'
33%|███▎ | 318/967 [04:09<08:48, 1.23it/s]
cannot identify image file './poster_small/0449869.jpg'
cannot identify image file './poster_small/0047526.jpg'
33%|███▎ | 320/967 [04:11<08:39, 1.25it/s]
cannot identify image file './poster_small/0095593.jpg'
33%|███▎ | 321/967 [04:12<08:17, 1.30it/s]
cannot identify image file './poster_small/2762334.jpg'
33%|███▎ | 322/967 [04:12<08:30, 1.26it/s]
cannot identify image file './poster_small/0023293.jpg'
34%|███▎ | 325/967 [04:15<08:01, 1.33it/s]
cannot identify image file './poster_small/0024593.jpg'
34%|███▍ | 327/967 [04:16<08:30, 1.25it/s]
cannot identify image file './poster_small/1116182.jpg'
34%|███▍ | 328/967 [04:17<08:58, 1.19it/s]
cannot identify image file './poster_small/0063462.jpg'
34%|███▍ | 329/967 [04:18<09:14, 1.15it/s]
cannot identify image file './poster_small/0119577.jpg'
34%|███▍ | 331/967 [04:19<07:48, 1.36it/s]
cannot identify image file './poster_small/0106727.jpg'
cannot identify image file './poster_small/0053884.jpg'
35%|███▍ | 337/967 [04:25<10:45, 1.03s/it]
cannot identify image file './poster_small/0037077.jpg'
35%|███▌ | 341/967 [04:29<10:09, 1.03it/s]
cannot identify image file './poster_small/0040064.jpg'
36%|███▌ | 345/967 [04:32<08:07, 1.28it/s]
cannot identify image file './poster_small/0089108.jpg'
36%|███▌ | 346/967 [04:33<08:30, 1.22it/s]
cannot identify image file './poster_small/0023129.jpg'
36%|███▌ | 347/967 [04:34<09:10, 1.13it/s]
cannot identify image file './poster_small/0044827.jpg'
37%|███▋ | 353/967 [04:39<09:09, 1.12it/s]
cannot identify image file './poster_small/0067108.jpg'
37%|███▋ | 359/967 [04:44<08:04, 1.25it/s]
cannot identify image file './poster_small/0432432.jpg'
37%|███▋ | 360/967 [04:45<09:17, 1.09it/s]
cannot identify image file './poster_small/0202415.jpg'
37%|███▋ | 362/967 [04:47<09:21, 1.08it/s]
cannot identify image file './poster_small/0074812.jpg'
38%|███▊ | 363/967 [04:48<09:48, 1.03it/s]
cannot identify image file './poster_small/0059311.jpg'
38%|███▊ | 371/967 [04:55<08:19, 1.19it/s]
cannot identify image file './poster_small/0065073.jpg'
39%|███▊ | 373/967 [04:56<07:01, 1.41it/s]
cannot identify image file './poster_small/0052820.jpg'
39%|███▉ | 375/967 [04:59<08:32, 1.15it/s]
cannot identify image file './poster_small/0120865.jpg'
39%|███▉ | 377/967 [05:01<09:15, 1.06it/s]
cannot identify image file './poster_small/0064620.jpg'
cannot identify image file './poster_small/0068505.jpg'
cannot identify image file './poster_small/2934916.jpg'
39%|███▉ | 379/967 [05:02<08:47, 1.11it/s]
cannot identify image file './poster_small/0040137.jpg'
40%|███▉ | 384/967 [05:07<09:01, 1.08it/s]
cannot identify image file './poster_small/0071864.jpg'
40%|███▉ | 385/967 [05:08<09:04, 1.07it/s]
cannot identify image file './poster_small/0072973.jpg'
40%|████ | 387/967 [05:09<07:45, 1.25it/s]
cannot identify image file './poster_small/0449951.jpg'
40%|████ | 388/967 [05:10<07:35, 1.27it/s]
cannot identify image file './poster_small/0074605.jpg'
40%|████ | 391/967 [05:13<08:07, 1.18it/s]
cannot identify image file './poster_small/0328955.jpg'
cannot identify image file './poster_small/0077294.jpg'
41%|████ | 393/967 [05:14<08:19, 1.15it/s]
cannot identify image file './poster_small/0987918.jpg'
41%|████ | 394/967 [05:15<07:44, 1.23it/s]
cannot identify image file './poster_small/0067520.jpg'
41%|████ | 395/967 [05:16<08:02, 1.19it/s]
cannot identify image file './poster_small/0220016.jpg'
41%|████ | 396/967 [05:17<08:38, 1.10it/s]
cannot identify image file './poster_small/0067236.jpg'
41%|████ | 397/967 [05:18<08:30, 1.12it/s]
cannot identify image file './poster_small/0085838.jpg'
41%|████ | 398/967 [05:19<08:39, 1.09it/s]
cannot identify image file './poster_small/0047561.jpg'
cannot identify image file './poster_small/0066075.jpg'
42%|████▏ | 407/967 [05:28<08:47, 1.06it/s]
cannot identify image file './poster_small/0123374.jpg'
42%|████▏ | 410/967 [05:31<08:57, 1.04it/s]
cannot identify image file './poster_small/0026143.jpg'
43%|████▎ | 411/967 [05:32<08:52, 1.04it/s]
cannot identify image file './poster_small/0064626.jpg'
43%|████▎ | 413/967 [05:33<07:47, 1.19it/s]
cannot identify image file './poster_small/0822388.jpg'
43%|████▎ | 420/967 [05:40<08:23, 1.09it/s]
cannot identify image file './poster_small/0101664.jpg'
44%|████▎ | 423/967 [05:42<07:53, 1.15it/s]
cannot identify image file './poster_small/0403579.jpg'
cannot identify image file './poster_small/0070112.jpg'
cannot identify image file './poster_small/2323633.jpg'
44%|████▍ | 427/967 [05:45<05:54, 1.52it/s]
cannot identify image file './poster_small/0203408.jpg'
44%|████▍ | 428/967 [05:45<05:28, 1.64it/s]
cannot identify image file './poster_small/1167638.jpg'
45%|████▍ | 432/967 [05:47<05:12, 1.71it/s]
cannot identify image file './poster_small/0144178.jpg'
45%|████▍ | 434/967 [05:49<06:01, 1.48it/s]
cannot identify image file './poster_small/0295432.jpg'
45%|████▍ | 435/967 [05:50<06:18, 1.41it/s]
cannot identify image file './poster_small/0123865.jpg'
45%|████▌ | 436/967 [05:50<05:42, 1.55it/s]
cannot identify image file './poster_small/0110530.jpg'
cannot identify image file './poster_small/0082817.jpg'
45%|████▌ | 437/967 [05:51<06:05, 1.45it/s]
cannot identify image file './poster_small/0067525.jpg'
45%|████▌ | 438/967 [05:52<06:34, 1.34it/s]
cannot identify image file './poster_small/0046333.jpg'
45%|████▌ | 439/967 [05:53<06:48, 1.29it/s]
cannot identify image file './poster_small/0248953.jpg'
46%|████▌ | 441/967 [05:55<07:57, 1.10it/s]
cannot identify image file './poster_small/0000033.jpg'
cannot identify image file './poster_small/0069165.jpg'
cannot identify image file './poster_small/0000014.jpg'
cannot identify image file './poster_small/0000027.jpg'
48%|████▊ | 460/967 [06:12<08:03, 1.05it/s]
cannot identify image file './poster_small/0063531.jpg'
48%|████▊ | 462/967 [06:14<07:04, 1.19it/s]
cannot identify image file './poster_small/0041431.jpg'
48%|████▊ | 463/967 [06:15<07:06, 1.18it/s]
cannot identify image file './poster_small/0831387.jpg'
48%|████▊ | 465/967 [06:16<07:06, 1.18it/s]
cannot identify image file './poster_small/3908598.jpg'
48%|████▊ | 467/967 [06:18<06:40, 1.25it/s]
cannot identify image file './poster_small/0056341.jpg'
49%|████▊ | 470/967 [06:21<07:14, 1.14it/s]
cannot identify image file './poster_small/3833520.jpg'
49%|████▉ | 472/967 [06:22<06:35, 1.25it/s]
cannot identify image file './poster_small/0058660.jpg'
49%|████▉ | 475/967 [06:24<06:05, 1.35it/s]
cannot identify image file './poster_small/0086847.jpg'
49%|████▉ | 476/967 [06:25<06:14, 1.31it/s]
cannot identify image file './poster_small/0074455.jpg'
49%|████▉ | 477/967 [06:26<06:57, 1.17it/s]
cannot identify image file './poster_small/0037990.jpg'
50%|████▉ | 481/967 [06:30<07:50, 1.03it/s]
cannot identify image file './poster_small/1764600.jpg'
50%|████▉ | 482/967 [06:31<07:57, 1.02it/s]
cannot identify image file './poster_small/0372764.jpg'
cannot identify image file './poster_small/0368576.jpg'
cannot identify image file './poster_small/0368574.jpg'
cannot identify image file './poster_small/0366178.jpg'
50%|█████ | 484/967 [06:33<07:24, 1.09it/s]
cannot identify image file './poster_small/0067118.jpg'
50%|█████ | 488/967 [06:36<06:22, 1.25it/s]
cannot identify image file './poster_small/0044954.jpg'
51%|█████▏ | 496/967 [06:42<06:30, 1.21it/s]
cannot identify image file './poster_small/0078950.jpg'
51%|█████▏ | 498/967 [06:44<06:36, 1.18it/s]
cannot identify image file './poster_small/0050957.jpg'
cannot identify image file './poster_small/0058374.jpg'
52%|█████▏ | 499/967 [06:45<06:23, 1.22it/s]
cannot identify image file './poster_small/0027963.jpg'
52%|█████▏ | 507/967 [06:52<07:13, 1.06it/s]
cannot identify image file './poster_small/0362590.jpg'
53%|█████▎ | 508/967 [06:53<06:47, 1.13it/s]
cannot identify image file './poster_small/0008309.jpg'
53%|█████▎ | 509/967 [06:54<06:37, 1.15it/s]
cannot identify image file './poster_small/0065240.jpg'
53%|█████▎ | 512/967 [06:57<07:17, 1.04it/s]
cannot identify image file './poster_small/0055022.jpg'
53%|█████▎ | 514/967 [06:59<07:04, 1.07it/s]
cannot identify image file './poster_small/0418753.jpg'
53%|█████▎ | 515/967 [07:00<06:59, 1.08it/s]
cannot identify image file './poster_small/0070768.jpg'
cannot identify image file './poster_small/1706680.jpg'
54%|█████▎ | 518/967 [07:02<06:11, 1.21it/s]
cannot identify image file './poster_small/3836530.jpg'
cannot identify image file './poster_small/0050545.jpg'
54%|█████▍ | 522/967 [07:04<04:37, 1.61it/s]
cannot identify image file './poster_small/8752440.jpg'
54%|█████▍ | 523/967 [07:05<04:04, 1.81it/s]
cannot identify image file './poster_small/0019504.jpg'
54%|█████▍ | 525/967 [07:06<05:05, 1.45it/s]
cannot identify image file './poster_small/0060117.jpg'
54%|█████▍ | 526/967 [07:07<05:27, 1.35it/s]
cannot identify image file './poster_small/1172060.jpg'
56%|█████▌ | 541/967 [07:19<05:28, 1.30it/s]
cannot identify image file './poster_small/3280916.jpg'
56%|█████▌ | 542/967 [07:20<05:17, 1.34it/s]
cannot identify image file './poster_small/0039502.jpg'
56%|█████▌ | 543/967 [07:21<06:04, 1.16it/s]
cannot identify image file './poster_small/3800796.jpg'
56%|█████▋ | 544/967 [07:22<06:33, 1.07it/s]
cannot identify image file './poster_small/0074238.jpg'
56%|█████▋ | 545/967 [07:23<06:10, 1.14it/s]
cannot identify image file './poster_small/0062032.jpg'
56%|█████▋ | 546/967 [07:24<05:56, 1.18it/s]
cannot identify image file './poster_small/0053891.jpg'
57%|█████▋ | 547/967 [07:24<05:38, 1.24it/s]
cannot identify image file './poster_small/0184115.jpg'
cannot identify image file './poster_small/0060968.jpg'
57%|█████▋ | 548/967 [07:25<05:44, 1.22it/s]
cannot identify image file './poster_small/0075165.jpg'
57%|█████▋ | 549/967 [07:26<05:25, 1.28it/s]
cannot identify image file './poster_small/0076998.jpg'
cannot identify image file './poster_small/0060176.jpg'
57%|█████▋ | 550/967 [07:27<05:28, 1.27it/s]
cannot identify image file './poster_small/0092745.jpg'
cannot identify image file './poster_small/0079936.jpg'
57%|█████▋ | 552/967 [07:28<04:52, 1.42it/s]
cannot identify image file './poster_small/0060747.jpg'
57%|█████▋ | 553/967 [07:29<04:53, 1.41it/s]
cannot identify image file './poster_small/2523756.jpg'
57%|█████▋ | 554/967 [07:29<05:16, 1.31it/s]
cannot identify image file './poster_small/0092217.jpg'
57%|█████▋ | 555/967 [07:31<06:02, 1.14it/s]
cannot identify image file './poster_small/0046906.jpg'
57%|█████▋ | 556/967 [07:31<06:00, 1.14it/s]
cannot identify image file './poster_small/0206226.jpg'
cannot identify image file './poster_small/0086484.jpg'
58%|█████▊ | 558/967 [07:33<05:56, 1.15it/s]
cannot identify image file './poster_small/0175471.jpg'
58%|█████▊ | 559/967 [07:34<06:12, 1.09it/s]
cannot identify image file './poster_small/0085913.jpg'
58%|█████▊ | 560/967 [07:35<06:22, 1.06it/s]
cannot identify image file './poster_small/0233687.jpg'
cannot identify image file './poster_small/0053214.jpg'
58%|█████▊ | 561/967 [07:36<06:37, 1.02it/s]
cannot identify image file './poster_small/0032794.jpg'
58%|█████▊ | 562/967 [07:37<06:28, 1.04it/s]
cannot identify image file './poster_small/0040765.jpg'
cannot identify image file './poster_small/0064541.jpg'
59%|█████▊ | 568/967 [07:42<05:06, 1.30it/s]
cannot identify image file './poster_small/0365109.jpg'
59%|█████▉ | 569/967 [07:43<05:12, 1.27it/s]
cannot identify image file './poster_small/0337721.jpg'
59%|█████▉ | 570/967 [07:43<05:16, 1.25it/s]
cannot identify image file './poster_small/0032234.jpg'
59%|█████▉ | 572/967 [07:45<05:13, 1.26it/s]
cannot identify image file './poster_small/0344604.jpg'
59%|█████▉ | 574/967 [07:47<05:47, 1.13it/s]
cannot identify image file './poster_small/0041349.jpg'
60%|██████ | 581/967 [07:52<04:12, 1.53it/s]
cannot identify image file './poster_small/0180073.jpg'
cannot identify image file './poster_small/6926486.jpg'
60%|██████ | 583/967 [07:54<04:51, 1.32it/s]
cannot identify image file './poster_small/0079596.jpg'
61%|██████ | 586/967 [07:58<07:17, 1.15s/it]
cannot identify image file './poster_small/0140603.jpg'
cannot identify image file './poster_small/0069745.jpg'
61%|██████ | 587/967 [07:59<07:07, 1.12s/it]
cannot identify image file './poster_small/0066154.jpg'
cannot identify image file './poster_small/1745787.jpg'
61%|██████ | 590/967 [08:02<05:57, 1.06it/s]
cannot identify image file './poster_small/0045995.jpg'
cannot identify image file './poster_small/0038675.jpg'
62%|██████▏ | 595/967 [08:06<05:36, 1.10it/s]
cannot identify image file './poster_small/0068971.jpg'
62%|██████▏ | 596/967 [08:07<05:42, 1.08it/s]
cannot identify image file './poster_small/0050205.jpg'
62%|██████▏ | 598/967 [08:09<05:45, 1.07it/s]
cannot identify image file './poster_small/0085175.jpg'
cannot identify image file './poster_small/0424237.jpg'
62%|██████▏ | 603/967 [08:14<06:38, 1.09s/it]
cannot identify image file './poster_small/0190524.jpg'
cannot identify image file './poster_small/3365778.jpg'
62%|██████▏ | 604/967 [08:15<06:19, 1.05s/it]
cannot identify image file './poster_small/8119752.jpg'
63%|██████▎ | 608/967 [08:23<10:15, 1.71s/it]
cannot identify image file './poster_small/0031742.jpg'
63%|██████▎ | 610/967 [08:25<09:16, 1.56s/it]
cannot identify image file './poster_small/0100465.jpg'
63%|██████▎ | 614/967 [08:31<08:09, 1.39s/it]
cannot identify image file './poster_small/0072097.jpg'
64%|██████▎ | 615/967 [08:32<08:29, 1.45s/it]
cannot identify image file './poster_small/0071771.jpg'
64%|██████▍ | 617/967 [08:35<07:18, 1.25s/it]
cannot identify image file './poster_small/0174997.jpg'
64%|██████▍ | 622/967 [08:39<05:10, 1.11it/s]
cannot identify image file './poster_small/0033676.jpg'
64%|██████▍ | 623/967 [08:40<04:56, 1.16it/s]
cannot identify image file './poster_small/0443567.jpg'
65%|██████▍ | 624/967 [08:41<04:51, 1.18it/s]
cannot identify image file './poster_small/0047559.jpg'
65%|██████▍ | 627/967 [08:44<05:15, 1.08it/s]
cannot identify image file './poster_small/0260295.jpg'
65%|██████▌ | 633/967 [08:49<05:07, 1.09it/s]
cannot identify image file './poster_small/0200768.jpg'
66%|██████▌ | 640/967 [08:54<04:37, 1.18it/s]
cannot identify image file './poster_small/0245238.jpg'
66%|██████▋ | 643/967 [08:57<04:31, 1.19it/s]
cannot identify image file './poster_small/0075679.jpg'
67%|██████▋ | 644/967 [08:58<04:37, 1.16it/s]
cannot identify image file './poster_small/0042418.jpg'
67%|██████▋ | 645/967 [09:00<07:22, 1.37s/it]
cannot identify image file './poster_small/0036814.jpg'
67%|██████▋ | 651/967 [09:05<04:30, 1.17it/s]
cannot identify image file './poster_small/0079756.jpg'
68%|██████▊ | 653/967 [09:07<04:54, 1.07it/s]
cannot identify image file './poster_small/0983922.jpg'
68%|██████▊ | 658/967 [09:11<04:42, 1.09it/s]
cannot identify image file './poster_small/0058642.jpg'
68%|██████▊ | 659/967 [09:12<05:02, 1.02it/s]
cannot identify image file './poster_small/0116016.jpg'
69%|██████▊ | 663/967 [09:15<03:52, 1.31it/s]
cannot identify image file './poster_small/0092238.jpg'
69%|██████▉ | 666/967 [09:18<04:22, 1.15it/s]
cannot identify image file './poster_small/2226519.jpg'
69%|██████▉ | 668/967 [09:20<04:30, 1.10it/s]
cannot identify image file './poster_small/0414982.jpg'
cannot identify image file './poster_small/0419641.jpg'
69%|██████▉ | 671/967 [09:23<04:19, 1.14it/s]
cannot identify image file './poster_small/0040246.jpg'
69%|██████▉ | 672/967 [09:24<04:46, 1.03it/s]
cannot identify image file './poster_small/0217168.jpg'
70%|██████▉ | 674/967 [09:25<04:19, 1.13it/s]
cannot identify image file './poster_small/0038452.jpg'
70%|██████▉ | 675/967 [09:26<04:07, 1.18it/s]
cannot identify image file './poster_small/3155242.jpg'
70%|███████ | 678/967 [09:29<04:14, 1.14it/s]
cannot identify image file './poster_small/0038255.jpg'
cannot identify image file './poster_small/0043153.jpg'
70%|███████ | 681/967 [09:31<03:52, 1.23it/s]
cannot identify image file './poster_small/0072209.jpg'
71%|███████ | 686/967 [09:36<04:12, 1.11it/s]
cannot identify image file './poster_small/0074797.jpg'
71%|███████ | 688/967 [09:37<03:30, 1.32it/s]
cannot identify image file './poster_small/2720826.jpg'
71%|███████▏ | 690/967 [09:38<03:04, 1.50it/s]
cannot identify image file './poster_small/0068227.jpg'
72%|███████▏ | 695/967 [09:42<03:32, 1.28it/s]
cannot identify image file './poster_small/0372765.jpg'
72%|███████▏ | 697/967 [09:44<03:39, 1.23it/s]
cannot identify image file './poster_small/0083713.jpg'
72%|███████▏ | 699/967 [09:45<03:18, 1.35it/s]
cannot identify image file './poster_small/0252133.jpg'
72%|███████▏ | 700/967 [09:46<03:15, 1.37it/s]
cannot identify image file './poster_small/0329913.jpg'
73%|███████▎ | 703/967 [09:48<03:06, 1.41it/s]
cannot identify image file './poster_small/0036840.jpg'
73%|███████▎ | 705/967 [09:49<02:47, 1.56it/s]
cannot identify image file './poster_small/0067956.jpg'
73%|███████▎ | 707/967 [09:50<02:33, 1.69it/s]
cannot identify image file './poster_small/2195566.jpg'
73%|███████▎ | 708/967 [09:51<02:58, 1.45it/s]
cannot identify image file './poster_small/0080549.jpg'
74%|███████▍ | 714/967 [09:56<03:08, 1.34it/s]
cannot identify image file './poster_small/0073398.jpg'
74%|███████▍ | 716/967 [09:58<03:08, 1.33it/s]
cannot identify image file './poster_small/0038205.jpg'
74%|███████▍ | 718/967 [09:59<03:32, 1.17it/s]
cannot identify image file './poster_small/0117220.jpg'
74%|███████▍ | 719/967 [10:00<03:35, 1.15it/s]
cannot identify image file './poster_small/0046198.jpg'
75%|███████▍ | 725/967 [10:06<03:44, 1.08it/s]
cannot identify image file './poster_small/0060351.jpg'
75%|███████▌ | 727/967 [10:07<02:57, 1.35it/s]
cannot identify image file './poster_small/0081568.jpg'
cannot identify image file './poster_small/0046921.jpg'
75%|███████▌ | 729/967 [10:08<02:44, 1.45it/s]
cannot identify image file './poster_small/0034739.jpg'
75%|███████▌ | 730/967 [10:09<02:35, 1.53it/s]
cannot identify image file './poster_small/0023251.jpg'
cannot identify image file './poster_small/0491764.jpg'
cannot identify image file './poster_small/0090642.jpg'
77%|███████▋ | 741/967 [10:16<02:41, 1.40it/s]
cannot identify image file './poster_small/0037928.jpg'
77%|███████▋ | 743/967 [10:18<02:48, 1.33it/s]
cannot identify image file './poster_small/0457430.jpg'
cannot identify image file './poster_small/0057283.jpg'
cannot identify image file './poster_small/0462519.jpg'
cannot identify image file './poster_small/0110546.jpg'
77%|███████▋ | 747/967 [10:21<02:58, 1.23it/s]
cannot identify image file './poster_small/0045197.jpg'
77%|███████▋ | 748/967 [10:22<02:50, 1.29it/s]
cannot identify image file './poster_small/0062523.jpg'
78%|███████▊ | 750/967 [10:23<02:53, 1.25it/s]
cannot identify image file './poster_small/0112454.jpg'
cannot identify image file './poster_small/0065243.jpg'
78%|███████▊ | 753/967 [10:25<02:14, 1.59it/s]
cannot identify image file './poster_small/0396171.jpg'
79%|███████▉ | 762/967 [10:32<02:34, 1.32it/s]
cannot identify image file './poster_small/0059710.jpg'
cannot identify image file './poster_small/0080928.jpg'
cannot identify image file './poster_small/0126004.jpg'
79%|███████▉ | 765/967 [10:34<02:34, 1.31it/s]
cannot identify image file './poster_small/1833116.jpg'
80%|███████▉ | 770/967 [10:38<02:02, 1.61it/s]
cannot identify image file './poster_small/0075766.jpg'
cannot identify image file './poster_small/0123860.jpg'
cannot identify image file './poster_small/0123970.jpg'
cannot identify image file './poster_small/0323120.jpg'
cannot identify image file './poster_small/0035301.jpg'
80%|███████▉ | 772/967 [10:39<02:26, 1.33it/s]
cannot identify image file './poster_small/1216520.jpg'
80%|███████▉ | 773/967 [10:40<02:42, 1.19it/s]
cannot identify image file './poster_small/0028331.jpg'
cannot identify image file './poster_small/1330015.jpg'
cannot identify image file './poster_small/0062443.jpg'
80%|████████ | 774/967 [10:41<02:53, 1.11it/s]
cannot identify image file './poster_small/0485241.jpg'
cannot identify image file './poster_small/0154467.jpg'
80%|████████ | 776/967 [10:43<02:57, 1.08it/s]
cannot identify image file './poster_small/5235348.jpg'
80%|████████ | 777/967 [10:44<02:57, 1.07it/s]
cannot identify image file './poster_small/0191074.jpg'
80%|████████ | 778/967 [10:45<02:56, 1.07it/s]
cannot identify image file './poster_small/0060168.jpg'
81%|████████ | 779/967 [10:46<02:45, 1.13it/s]
cannot identify image file './poster_small/0081738.jpg'
81%|████████ | 782/967 [10:48<02:13, 1.39it/s]
cannot identify image file './poster_small/0379473.jpg'
81%|████████▏ | 788/967 [10:53<02:33, 1.17it/s]
cannot identify image file './poster_small/0063381.jpg'
cannot identify image file './poster_small/4427076.jpg'
cannot identify image file './poster_small/0173714.jpg'
82%|████████▏ | 791/967 [10:56<02:28, 1.19it/s]
cannot identify image file './poster_small/3794028.jpg'
82%|████████▏ | 793/967 [10:57<02:32, 1.14it/s]
cannot identify image file './poster_small/0464106.jpg'
cannot identify image file './poster_small/0090570.jpg'
82%|████████▏ | 794/967 [10:58<02:27, 1.18it/s]
cannot identify image file './poster_small/0087829.jpg'
83%|████████▎ | 799/967 [11:01<01:39, 1.69it/s]
cannot identify image file './poster_small/0041866.jpg'
83%|████████▎ | 802/967 [11:03<02:02, 1.35it/s]
cannot identify image file './poster_small/0444682.jpg'
cannot identify image file './poster_small/0058110.jpg'
83%|████████▎ | 805/967 [11:06<02:19, 1.16it/s]
cannot identify image file './poster_small/0072392.jpg'
cannot identify image file './poster_small/0080546.jpg'
83%|████████▎ | 806/967 [11:07<02:31, 1.06it/s]
cannot identify image file './poster_small/0064482.jpg'
84%|████████▎ | 809/967 [11:10<02:19, 1.13it/s]
cannot identify image file './poster_small/0044599.jpg'
84%|████████▍ | 812/967 [11:12<01:59, 1.29it/s]
cannot identify image file './poster_small/0439771.jpg'
84%|████████▍ | 814/967 [11:13<01:36, 1.58it/s]
cannot identify image file './poster_small/0021756.jpg'
85%|████████▍ | 820/967 [11:19<02:30, 1.02s/it]
cannot identify image file './poster_small/0039676.jpg'
cannot identify image file './poster_small/0160801.jpg'
85%|████████▍ | 821/967 [11:20<02:19, 1.04it/s]
cannot identify image file './poster_small/0032981.jpg'
cannot identify image file './poster_small/0049854.jpg'
85%|████████▌ | 823/967 [11:22<02:17, 1.05it/s]
cannot identify image file './poster_small/2605312.jpg'
cannot identify image file './poster_small/0367257.jpg'
86%|████████▌ | 829/967 [11:27<02:04, 1.11it/s]
cannot identify image file './poster_small/6817944.jpg'
87%|████████▋ | 839/967 [11:36<01:39, 1.28it/s]
cannot identify image file './poster_small/0082081.jpg'
87%|████████▋ | 841/967 [11:37<01:19, 1.59it/s]
cannot identify image file './poster_small/1146283.jpg'
cannot identify image file './poster_small/0796335.jpg'
87%|████████▋ | 842/967 [11:38<01:34, 1.32it/s]
cannot identify image file './poster_small/0183355.jpg'
87%|████████▋ | 844/967 [11:40<01:45, 1.17it/s]
cannot identify image file './poster_small/0218094.jpg'
cannot identify image file './poster_small/0290820.jpg'
87%|████████▋ | 845/967 [11:41<01:43, 1.18it/s]
cannot identify image file './poster_small/1059793.jpg'
cannot identify image file './poster_small/0025665.jpg'
88%|████████▊ | 848/967 [11:45<02:11, 1.11s/it]
cannot identify image file './poster_small/0259786.jpg'
88%|████████▊ | 854/967 [11:50<01:36, 1.17it/s]
cannot identify image file './poster_small/0044369.jpg'
88%|████████▊ | 855/967 [11:51<01:36, 1.16it/s]
cannot identify image file './poster_small/0031127.jpg'
89%|████████▊ | 857/967 [11:52<01:35, 1.16it/s]
cannot identify image file './poster_small/0283644.jpg'
89%|████████▉ | 860/967 [11:55<01:32, 1.16it/s]
cannot identify image file './poster_small/0316599.jpg'
90%|████████▉ | 866/967 [12:00<01:23, 1.21it/s]
cannot identify image file './poster_small/0118767.jpg'
90%|████████▉ | 867/967 [12:01<01:11, 1.39it/s]
cannot identify image file './poster_small/0059758.jpg'
cannot identify image file './poster_small/0122194.jpg'
90%|████████▉ | 868/967 [12:02<01:15, 1.32it/s]
cannot identify image file './poster_small/0070404.jpg'
90%|████████▉ | 869/967 [12:02<01:15, 1.30it/s]
cannot identify image file './poster_small/0028484.jpg'
90%|████████▉ | 870/967 [12:03<01:18, 1.24it/s]
cannot identify image file './poster_small/0166792.jpg'
cannot identify image file './poster_small/0369903.jpg'
91%|█████████ | 880/967 [12:12<01:05, 1.33it/s]
cannot identify image file './poster_small/0073115.jpg'
91%|█████████▏| 884/967 [12:15<01:08, 1.21it/s]
cannot identify image file './poster_small/0284655.jpg'
92%|█████████▏| 885/967 [12:16<01:06, 1.23it/s]
cannot identify image file './poster_small/9236264.jpg'
92%|█████████▏| 892/967 [12:22<01:03, 1.18it/s]
cannot identify image file './poster_small/0137094.jpg'
92%|█████████▏| 893/967 [12:23<00:59, 1.25it/s]
cannot identify image file './poster_small/0064323.jpg'
93%|█████████▎| 895/967 [12:24<00:48, 1.49it/s]
cannot identify image file './poster_small/0062741.jpg'
93%|█████████▎| 897/967 [12:25<00:41, 1.68it/s]
cannot identify image file './poster_small/0084237.jpg'
93%|█████████▎| 901/967 [12:28<00:48, 1.35it/s]
cannot identify image file './poster_small/0084273.jpg'
93%|█████████▎| 903/967 [12:30<00:50, 1.27it/s]
cannot identify image file './poster_small/4193400.jpg'
94%|█████████▎| 906/967 [12:32<00:50, 1.21it/s]
cannot identify image file './poster_small/0124307.jpg'
94%|█████████▍| 908/967 [12:34<00:51, 1.14it/s]
cannot identify image file './poster_small/0157383.jpg'
94%|█████████▍| 913/967 [12:37<00:35, 1.53it/s]
cannot identify image file './poster_small/0412808.jpg'
95%|█████████▍| 915/967 [12:39<00:35, 1.48it/s]
cannot identify image file './poster_small/0161860.jpg'
95%|█████████▍| 918/967 [12:41<00:31, 1.58it/s]
cannot identify image file './poster_small/4613254.jpg'
95%|█████████▌| 920/967 [12:42<00:32, 1.44it/s]
cannot identify image file './poster_small/2788556.jpg'
96%|█████████▌| 925/967 [12:46<00:34, 1.21it/s]
cannot identify image file './poster_small/1437361.jpg'
96%|█████████▌| 926/967 [12:47<00:34, 1.19it/s]
cannot identify image file './poster_small/3037582.jpg'
96%|█████████▌| 928/967 [12:49<00:29, 1.32it/s]
cannot identify image file './poster_small/0048211.jpg'
97%|█████████▋| 937/967 [12:57<00:26, 1.13it/s]
cannot identify image file './poster_small/4516162.jpg'
cannot identify image file './poster_small/0033932.jpg'
97%|█████████▋| 942/967 [13:00<00:17, 1.43it/s]
cannot identify image file './poster_small/0042871.jpg'
98%|█████████▊| 943/967 [13:01<00:15, 1.57it/s]
cannot identify image file './poster_small/0137799.jpg'
98%|█████████▊| 944/967 [13:01<00:14, 1.62it/s]
cannot identify image file './poster_small/1714196.jpg'
98%|█████████▊| 945/967 [13:02<00:14, 1.53it/s]
cannot identify image file './poster_small/0025117.jpg'
98%|█████████▊| 946/967 [13:03<00:13, 1.51it/s]
cannot identify image file './poster_small/2357144.jpg'
98%|█████████▊| 948/967 [13:04<00:12, 1.53it/s]
cannot identify image file './poster_small/1525898.jpg'
cannot identify image file './poster_small/0098088.jpg'
98%|█████████▊| 950/967 [13:06<00:13, 1.24it/s]
cannot identify image file './poster_small/6537238.jpg'
cannot identify image file './poster_small/0303151.jpg'
98%|█████████▊| 951/967 [13:07<00:13, 1.22it/s]
cannot identify image file './poster_small/0315632.jpg'
99%|█████████▊| 953/967 [13:09<00:12, 1.16it/s]
cannot identify image file './poster_small/0316352.jpg'
99%|█████████▉| 955/967 [13:11<00:11, 1.08it/s]
cannot identify image file './poster_small/0166557.jpg'
99%|█████████▉| 956/967 [13:12<00:10, 1.06it/s]
cannot identify image file './poster_small/0066879.jpg'
99%|█████████▉| 957/967 [13:13<00:09, 1.04it/s]
cannot identify image file './poster_small/3736766.jpg'
99%|█████████▉| 958/967 [13:13<00:08, 1.11it/s]
cannot identify image file './poster_small/0140340.jpg'
99%|█████████▉| 959/967 [13:14<00:06, 1.27it/s]
cannot identify image file './poster_small/1570970.jpg'
cannot identify image file './poster_small/0075364.jpg'
100%|█████████▉| 966/967 [13:21<00:01, 1.07s/it]
cannot identify image file './poster_small/0099901.jpg'
100%|██████████| 967/967 [13:23<00:00, 1.20it/s]
Unable to extract features for 447 images
import pickle
with open('movies_poster_features.pkl', 'wb') as f:
pickle.dump({"feature_dict": feature_dict}, f, protocol=pickle.HIGHEST_PROTOCOL)
len(feature_dict)
61504