# Copyright 2022 NVIDIA Corporation. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
# Each user is responsible for checking the content of datasets and the
# applicable licenses and determining if suitable for the intended use.
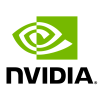
End-to-end session-based recommendations with PyTorch
In recent years, several deep learning-based algorithms have been proposed for recommendation systems while its adoption in industry deployments have been steeply growing. In particular, NLP inspired approaches have been successfully adapted for sequential and session-based recommendation problems, which are important for many domains like e-commerce, news and streaming media. Session-Based Recommender Systems (SBRS) have been proposed to model the sequence of interactions within the current user session, where a session is a short sequence of user interactions typically bounded by user inactivity. They have recently gained popularity due to their ability to capture short-term or contextual user preferences towards items.
The field of NLP has evolved significantly within the last decade, particularly due to the increased usage of deep learning. As a result, state of the art NLP approaches have inspired RecSys practitioners and researchers to adapt those architectures, especially for sequential and session-based recommendation problems. Here, we leverage one of the state-of-the-art Transformer-based architecture, XLNet with Masked Language Modeling (MLM) training technique (see our tutorial for details) for training a session-based model.
In this end-to-end-session-based recommnender model example, we use Transformers4Rec
library, which leverages the popular HuggingFace’s Transformers NLP library and make it possible to experiment with cutting-edge implementation of such architectures for sequential and session-based recommendation problems. For detailed explanations of the building blocks of Transformers4Rec meta-architecture visit getting-started-session-based and tutorial example notebooks.
1. Model definition using Transformers4Rec
In the previous notebook, we have created sequential features and saved our processed data frames as parquet files. Now we use these processed parquet files to train a session-based recommendation model with the XLNet architecture.
1.1 Get the schema
The library uses a schema format to configure the input features and automatically creates the necessary layers. This protobuf text file contains the description of each input feature by defining: the name, the type, the number of elements of a list column, the cardinality of a categorical feature and the min and max values of each feature. In addition, the annotation field contains the tags such as specifying the continuous
and categorical
features, the target
column or the item_id
feature, among others.
We create the schema object by reading the processed train parquet file generated by NVTabular pipeline in the previous, 01-ETL-with-NVTabular, notebook.
import os
os.environ["CUDA_VISIBLE_DEVICES"]="0"
INPUT_DATA_DIR = os.environ.get("INPUT_DATA_DIR", "/workspace/data")
OUTPUT_DIR = os.environ.get("OUTPUT_DIR", f"{INPUT_DATA_DIR}/preproc_sessions_by_day")
from merlin.schema import Schema
from merlin.io import Dataset
train = Dataset(os.path.join(INPUT_DATA_DIR, "processed_nvt/part_0.parquet"))
schema = train.schema
/usr/local/lib/python3.8/dist-packages/merlin/dtypes/mappings/tf.py:52: UserWarning: Tensorflow dtype mappings did not load successfully due to an error: No module named 'tensorflow'
warn(f"Tensorflow dtype mappings did not load successfully due to an error: {exc.msg}")
We can select the subset of features we want to use for training the model by their tags or their names.
schema = schema.select_by_name(
['item_id-list', 'category-list', 'product_recency_days_log_norm-list', 'et_dayofweek_sin-list']
)
We can print out the schema.
schema
name | tags | dtype | is_list | is_ragged | properties.num_buckets | properties.freq_threshold | properties.max_size | properties.cat_path | properties.embedding_sizes.cardinality | properties.embedding_sizes.dimension | properties.domain.min | properties.domain.max | properties.domain.name | properties.value_count.min | properties.value_count.max | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | item_id-list | (Tags.CATEGORICAL, Tags.LIST, Tags.ID, Tags.ITEM) | DType(name='int64', element_type=<ElementType.... | True | True | NaN | 0.0 | 0.0 | .//categories/unique.item_id.parquet | 52742.0 | 512.0 | 0.0 | 52741.0 | item_id | 0 | 20 |
1 | category-list | (Tags.CATEGORICAL, Tags.LIST) | DType(name='int64', element_type=<ElementType.... | True | True | NaN | 0.0 | 0.0 | .//categories/unique.category.parquet | 337.0 | 42.0 | 0.0 | 336.0 | category | 0 | 20 |
2 | product_recency_days_log_norm-list | (Tags.LIST, Tags.CONTINUOUS) | DType(name='float32', element_type=<ElementTyp... | True | True | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | 0 | 20 |
3 | et_dayofweek_sin-list | (Tags.LIST, Tags.CONTINUOUS) | DType(name='float64', element_type=<ElementTyp... | True | True | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | 0 | 20 |
1.2 Define the end-to-end Session-based Transformer-based recommendation model
For defining a session-based recommendation model, the end-to-end model definition requires four steps:
Instantiate TabularSequenceFeatures input-module from schema to prepare the embedding tables of categorical variables and project continuous features, if specified. In addition, the module provides different aggregation methods (e.g. ‘concat’, ‘elementwise-sum’) to merge input features and generate the sequence of interactions embeddings. The module also supports language modeling tasks to prepare masked labels for training and evaluation (e.g: ‘mlm’ for masked language modeling)
Next, we need to define one or multiple prediction tasks. For this demo, we are going to use NextItemPredictionTask with
Masked Language modeling
: during training, randomly selected items are masked and predicted using the unmasked sequence items. For inference, it is meant to always predict the next item to be interacted with.Then we construct a
transformer_config
based on the architectures provided by Hugging Face Transformers framework.Finally we link the transformer-body to the inputs and the prediction tasks to get the final pytorch
Model
class.
For more details about the features supported by each sub-module, please check out the library documentation page.
from transformers4rec import torch as tr
max_sequence_length, d_model = 20, 320
# Define input module to process tabular input-features and to prepare masked inputs
input_module = tr.TabularSequenceFeatures.from_schema(
schema,
max_sequence_length=max_sequence_length,
continuous_projection=64,
aggregation="concat",
d_output=d_model,
masking="mlm",
)
# Define Next item prediction-task
prediction_task = tr.NextItemPredictionTask(weight_tying=True)
# Define the config of the XLNet Transformer architecture
transformer_config = tr.XLNetConfig.build(
d_model=d_model, n_head=8, n_layer=2, total_seq_length=max_sequence_length
)
# Get the end-to-end model
model = transformer_config.to_torch_model(input_module, prediction_task)
/usr/local/lib/python3.8/dist-packages/tqdm/auto.py:21: TqdmWarning: IProgress not found. Please update jupyter and ipywidgets. See https://ipywidgets.readthedocs.io/en/stable/user_install.html
from .autonotebook import tqdm as notebook_tqdm
Projecting inputs of NextItemPredictionTask to'64' As weight tying requires the input dimension '320' to be equal to the item-id embedding dimension '64'
You can print out the model structure by uncommenting the line below.
#model
1.3. Daily Fine-Tuning: Training over a time window¶
Now that the model is defined, we are going to launch training. For that, Transfromers4rec extends HF Transformers Trainer class to adapt the evaluation loop for session-based recommendation task and the calculation of ranking metrics. The original train()
method is not modified meaning that we leverage the efficient training implementation from that library, which manages, for example, half-precision (FP16) training.
Set the training arguments
An additional argument data_loader_engine
is defined to automatically load the features needed for training using the schema. The default value is merlin
for optimized GPU-based data-loading. Optionally a PyarrowDataLoader
(pyarrow
) can also be used as a basic option, but it is slower and works only for small datasets, as the full data is loaded to CPU memory.
BATCH_SIZE_TRAIN = int(os.environ.get("BATCH_SIZE_TRAIN", "512"))
BATCH_SIZE_VALID = int(os.environ.get("BATCH_SIZE_VALID", "256"))
training_args = tr.trainer.T4RecTrainingArguments(
output_dir="./tmp",
max_sequence_length=20,
data_loader_engine='merlin',
num_train_epochs=10,
dataloader_drop_last=False,
per_device_train_batch_size = BATCH_SIZE_TRAIN,
per_device_eval_batch_size = BATCH_SIZE_VALID,
learning_rate=0.0005,
fp16=True,
report_to = [],
logging_steps=200
)
Instantiate the trainer
recsys_trainer = tr.Trainer(
model=model,
args=training_args,
schema=schema,
compute_metrics=True)
Using amp fp16 backend
Launch daily training and evaluation
In this demo, we will use the fit_and_evaluate
method that allows us to conduct a time-based finetuning by iteratively training and evaluating using a sliding time window: At each iteration, we use the training data of a specific time index \(t\) to train the model; then we evaluate on the validation data of the next index \(t + 1\). Particularly, we set start time to 178 and end time to 180.
If you have generated a synthetic dataset in the previous notebook, remember to change the values 178 and 180 accordingly.
from transformers4rec.torch.utils.examples_utils import fit_and_evaluate
start_time_idx = int(os.environ.get("START_TIME_INDEX", "178"))
end_time_idx = int(os.environ.get("END_TIME_INDEX", "180"))
OT_results = fit_and_evaluate(recsys_trainer, start_time_index=start_time_idx, end_time_index=end_time_idx, input_dir=OUTPUT_DIR)
***** Launch training for day 178: *****
***** Running training *****
Num examples = 28672
Num Epochs = 10
Instantaneous batch size per device = 512
Total train batch size (w. parallel, distributed & accumulation) = 512
Gradient Accumulation steps = 1
Total optimization steps = 560
Step | Training Loss |
---|---|
200 | 7.585600 |
400 | 6.608700 |
Saving model checkpoint to ./tmp/checkpoint-500
Trainer.model is not a `PreTrainedModel`, only saving its state dict.
Training completed. Do not forget to share your model on huggingface.co/models =)
***** Running training *****
Num examples = 20480
Num Epochs = 10
Instantaneous batch size per device = 512
Total train batch size (w. parallel, distributed & accumulation) = 512
Gradient Accumulation steps = 1
Total optimization steps = 400
***** Evaluation results for day 179:*****
eval_/next-item/avg_precision@10 = 0.07277625054121017
eval_/next-item/avg_precision@20 = 0.077287457883358
eval_/next-item/ndcg@10 = 0.1008271649479866
eval_/next-item/ndcg@20 = 0.11763089150190353
eval_/next-item/recall@10 = 0.18959537148475647
eval_/next-item/recall@20 = 0.25549131631851196
***** Launch training for day 179: *****
Step | Training Loss |
---|---|
200 | 6.838400 |
400 | 6.304600 |
Training completed. Do not forget to share your model on huggingface.co/models =)
***** Running training *****
Num examples = 16896
Num Epochs = 10
Instantaneous batch size per device = 512
Total train batch size (w. parallel, distributed & accumulation) = 512
Gradient Accumulation steps = 1
Total optimization steps = 330
***** Evaluation results for day 180:*****
eval_/next-item/avg_precision@10 = 0.059328265488147736
eval_/next-item/avg_precision@20 = 0.06352042406797409
eval_/next-item/ndcg@10 = 0.08318208903074265
eval_/next-item/ndcg@20 = 0.09845318645238876
eval_/next-item/recall@10 = 0.16083915531635284
eval_/next-item/recall@20 = 0.2209790199995041
***** Launch training for day 180: *****
Step | Training Loss |
---|---|
200 | 6.708000 |
Training completed. Do not forget to share your model on huggingface.co/models =)
***** Evaluation results for day 181:*****
eval_/next-item/avg_precision@10 = 0.12736327946186066
eval_/next-item/avg_precision@20 = 0.13500627875328064
eval_/next-item/ndcg@10 = 0.16738776862621307
eval_/next-item/ndcg@20 = 0.19680777192115784
eval_/next-item/recall@10 = 0.29406309127807617
eval_/next-item/recall@20 = 0.41187384724617004
Visualize the average of metrics over time
OT_results
is a list of scores (accuracy metrics) for evaluation based on given start and end time_index. Since in this example we do evaluation on days 179, 180 and 181, we get three metrics in the list one for each day.
OT_results
{'indexed_by_time_eval_/next-item/avg_precision@10': [0.07277625054121017,
0.059328265488147736,
0.12736327946186066],
'indexed_by_time_eval_/next-item/avg_precision@20': [0.077287457883358,
0.06352042406797409,
0.13500627875328064],
'indexed_by_time_eval_/next-item/ndcg@10': [0.1008271649479866,
0.08318208903074265,
0.16738776862621307],
'indexed_by_time_eval_/next-item/ndcg@20': [0.11763089150190353,
0.09845318645238876,
0.19680777192115784],
'indexed_by_time_eval_/next-item/recall@10': [0.18959537148475647,
0.16083915531635284,
0.29406309127807617],
'indexed_by_time_eval_/next-item/recall@20': [0.25549131631851196,
0.2209790199995041,
0.41187384724617004]}
import numpy as np
# take the average of metric values over time
avg_results = {k: np.mean(v) for k,v in OT_results.items()}
for key in sorted(avg_results.keys()):
print(" %s = %s" % (key, str(avg_results[key])))
indexed_by_time_eval_/next-item/avg_precision@10 = 0.08648926516373952
indexed_by_time_eval_/next-item/avg_precision@20 = 0.09193805356820424
indexed_by_time_eval_/next-item/ndcg@10 = 0.1171323408683141
indexed_by_time_eval_/next-item/ndcg@20 = 0.13763061662515005
indexed_by_time_eval_/next-item/recall@10 = 0.21483253935972849
indexed_by_time_eval_/next-item/recall@20 = 0.2961147278547287
Trace the model
We serve the model with the PyTorch backend that is used to execute TorchScript models. All models created in PyTorch using the python API must be traced/scripted to produce a TorchScript model. For tracing the model, we use torch.jit.trace api that takes the model as a Python function or torch.nn.Module, and an example input that will be passed to the function while tracing.
import os
import torch
import cudf
from merlin.io import Dataset
from nvtabular import Workflow
from merlin.systems.dag import Ensemble
from merlin.systems.dag.ops.pytorch import PredictPyTorch
from merlin.systems.dag.ops.workflow import TransformWorkflow
from merlin.table import TensorTable, TorchColumn
from merlin.table.conversions import convert_col
Create a dict of tensors to feed it as example inputs in the torch.jit.trace()
.
df = cudf.read_parquet(os.path.join(INPUT_DATA_DIR, f"preproc_sessions_by_day/{start_time_idx}/train.parquet"), columns=model.input_schema.column_names)
table = TensorTable.from_df(df.iloc[:100])
for column in table.columns:
table[column] = convert_col(table[column], TorchColumn)
model_input_dict = table.to_dict()
Let’s now add a top_k parameter to model so that we can return the top k item ids with the highest scores when we serve the model on Triton Inference Server.
topk = 20
model.top_k = topk
Let us now trace the model.
model.eval()
traced_model = torch.jit.trace(model, model_input_dict, strict=True)
Let’s check out the item_id-list
column in the model_input_dict
dictionary.
The column is represented as values and offsets dictating which values belong to which example.
model_input_dict['item_id-list__values']
tensor([ 605, 879, 743, 91, 4778, 1584, 3447, 8084, 3447, 4019,
743, 4778, 185, 12289, 2066, 431, 7, 31, 7, 158,
1988, 2591, 10856, 8218, 4211, 8712, 4243, 82, 113, 4243,
5733, 6811, 34, 7, 74, 665, 2313, 7125, 9114, 446,
1158, 775, 686, 431, 1946, 476, 598, 290, 167, 30,
343, 290, 34, 424, 167, 481, 2773, 289, 963, 4002,
2051, 3275, 500, 1220, 396, 1637, 11840, 10715, 11108, 290,
167, 651, 1086, 303, 89, 651, 215, 305, 178, 318,
424, 7, 3819, 932, 2187, 1086, 207, 688, 3832, 688,
203, 21, 44, 21, 2035, 10458, 21, 22, 1127, 2816,
4211, 34265, 831, 775, 621, 2051, 1988, 1080, 4714, 1337,
662, 290, 431, 864, 4830, 5787, 19157, 17271, 23366, 4210,
3652, 1038, 4771, 225, 278, 1021, 651, 167, 1355, 207,
1890, 2474, 1698, 998, 481, 775, 3842, 4317, 3842, 1231,
3842, 1698, 3810, 476, 982, 805, 314, 614, 1220, 1335,
1942, 2889, 2627, 1335, 2690, 805, 476, 982, 314, 805,
1220, 207, 652, 430, 606, 102, 414, 1966, 628, 815,
628, 815, 4714, 3676, 2790, 3770, 1284, 9541, 1252, 314,
686, 2498, 396, 846, 3463, 2714, 5078, 389, 341, 298,
389, 9642, 47, 62, 823, 62, 603, 2271, 720, 3275,
2557, 3, 62, 25, 97, 62, 424, 62, 476, 3461,
2694, 3461, 3045, 2557, 3989, 993, 1604, 123, 2705, 2788,
3136, 551, 517, 45, 1552, 2703, 207, 1763, 32, 475,
482, 199, 475, 2705, 2394, 1026, 20034, 73, 1335, 225,
3461, 4775, 2051, 6486, 15954, 423, 1489, 2347, 4471, 2549,
572, 1771, 1325, 454, 838, 124, 639, 4760, 3553, 6826,
2741, 5348, 5391, 1170, 4101, 1231, 805, 3589, 2450, 186,
17, 644, 275, 687, 18093, 10458, 610, 2970, 3481, 2970,
38, 610, 2970, 610, 23, 8313, 258, 38, 23, 5362,
7187, 7381, 6053, 7257, 13405, 558, 161, 1665, 4376, 3485,
686, 652, 446, 430, 446, 775, 652, 4285, 1739, 3856,
226, 211, 7246, 6732, 772, 1988, 158, 805, 443, 805,
2092, 1170, 2092, 1170, 3485, 4376, 3485, 446, 430, 431,
424, 1698, 1394, 1799, 2754, 207, 1154, 21589, 2190, 3705,
4464, 5817, 7558, 508, 1798, 815, 628, 2017, 856, 1890,
225, 598, 38, 598, 16534, 10256, 3, 2652, 4029, 2557,
2789, 6380, 1831, 1071, 31, 313, 446, 1086, 1570, 2223,
2665, 1951, 2099, 1673, 225, 4337, 652, 998, 1158, 831,
801, 598, 1086, 12431, 416, 12431, 652, 801, 1757, 1379,
1414, 634, 2035, 7933, 6035, 6361, 4663, 577, 4663, 577,
4663], device='cuda:0')
And here are the offsets
model_input_dict['item_id-list__offsets']
tensor([ 0, 12, 14, 16, 19, 21, 23, 26, 32, 35, 45, 47, 49, 51,
56, 59, 61, 66, 69, 72, 76, 78, 80, 83, 87, 91, 94, 96,
98, 100, 102, 104, 107, 111, 113, 122, 124, 128, 130, 133, 136, 141,
143, 161, 164, 167, 172, 176, 180, 182, 184, 191, 193, 196, 199, 201,
209, 214, 234, 237, 243, 245, 265, 267, 274, 276, 281, 289, 295, 298,
300, 305, 307, 312, 314, 317, 320, 324, 327, 331, 335, 337, 339, 343,
345, 348, 351, 354, 356, 360, 362, 364, 367, 374, 376, 381, 383, 386,
388, 396, 401], device='cuda:0', dtype=torch.int32)
Let’s create a folder that we can store the exported models and the config files.
import shutil
ens_model_path = os.environ.get("ens_model_path", f"{INPUT_DATA_DIR}/models")
# Make sure we have a clean stats space for Dask
if os.path.isdir(ens_model_path):
shutil.rmtree(ens_model_path)
os.mkdir(ens_model_path)
workflow = Workflow.load(os.path.join(INPUT_DATA_DIR, "workflow_etl"))
torch_op = workflow.input_schema.column_names >> TransformWorkflow(workflow) >> PredictPyTorch(
traced_model, model.input_schema, model.output_schema
)
The last step is to create the ensemble artifacts that Triton Inference Server can consume. To make these artifacts, we import the Ensemble class. The class is responsible for interpreting the graph and exporting the correct files for the server.
When we create an Ensemble
object we supply the graph and a schema representing the starting input of the graph. The inputs to the ensemble graph are the inputs to the first operator of out graph. After we created the Ensemble we export the graph, supplying an export path for the ensemble.export
function. This returns an ensemble config which represents the entire inference pipeline and a list of node-specific configs.
ensemble = Ensemble(torch_op, workflow.input_schema)
ens_config, node_configs = ensemble.export(ens_model_path)
/usr/local/lib/python3.8/dist-packages/merlin/systems/dag/node.py:100: UserWarning: Operator 'TransformWorkflow' is producing the output column 'session_id', which is not being used by any downstream operator in the ensemble graph.
warnings.warn(
/usr/local/lib/python3.8/dist-packages/merlin/systems/dag/node.py:100: UserWarning: Operator 'TransformWorkflow' is producing the output column 'item_id-count', which is not being used by any downstream operator in the ensemble graph.
warnings.warn(
/usr/local/lib/python3.8/dist-packages/merlin/systems/dag/node.py:100: UserWarning: Operator 'TransformWorkflow' is producing the output column 'day_index', which is not being used by any downstream operator in the ensemble graph.
warnings.warn(
2. Serving Ensemble Model to the Triton Inference Server
NVIDIA Triton Inference Server (TIS) simplifies the deployment of AI models at scale in production. TIS provides a cloud and edge inferencing solution optimized for both CPUs and GPUs. It supports a number of different machine learning frameworks such as TensorFlow and PyTorch.
The last step of a machine learning (ML)/deep learning (DL) pipeline is to deploy the ETL workflow and saved model to production. In the production setting, we want to transform the input data as done during training (ETL). We need to apply the same mean/std for continuous features and use the same categorical mapping to convert the categories to continuous integer before we use the DL model for a prediction. Therefore, we deploy the NVTabular workflow with the PyTorch model as an ensemble model to Triton Inference. The ensemble model guarantees that the same transformation is applied to the raw inputs.
In this section, you will learn how to
to deploy saved NVTabular and PyTorch models to Triton Inference Server
send requests for predictions and get responses.
2.1 Starting Triton Server
It is time to deploy all the models as an ensemble model to Triton Inference Serve TIS. After we export the ensemble, we are ready to start the TIS. You can start triton server by using the following command on your terminal:
tritonserver --model-repository=<ensemble_export_path>
For the --model-repository
argument, specify the same path as the export_path that you specified previously in the ensemble.export
method. This command will launch the server and load all the models to the server. Once all the models are loaded successfully, you should see READY status printed out in the terminal for each loaded model.
2.2. Connect to the Triton Inference Server and check if the server is alive
import tritonhttpclient
try:
triton_client = tritonhttpclient.InferenceServerClient(url="localhost:8000", verbose=True)
print("client created.")
except Exception as e:
print("channel creation failed: " + str(e))
triton_client.is_server_live()
client created.
GET /v2/health/live, headers None
<HTTPSocketPoolResponse status=200 headers={'content-length': '0', 'content-type': 'text/plain'}>
/usr/local/lib/python3.8/dist-packages/tritonhttpclient/__init__.py:31: DeprecationWarning: The package `tritonhttpclient` is deprecated and will be removed in a future version. Please use instead `tritonclient.http`
warnings.warn(
True
2.3. Load raw data for inference
We select the last 50 interactions and filter out sessions with less than 2 interactions.
import pandas as pd
interactions_merged_df = pd.read_parquet(os.path.join(INPUT_DATA_DIR, "interactions_merged_df.parquet"))
interactions_merged_df = interactions_merged_df.sort_values('timestamp')
batch = interactions_merged_df[-50:]
sessions_to_use = batch.session_id.value_counts()
filtered_batch = batch[batch.session_id.isin(sessions_to_use[sessions_to_use.values>1].index.values)]
2.5. Send the request to triton server
triton_client.get_model_repository_index()
POST /v2/repository/index, headers None
<HTTPSocketPoolResponse status=200 headers={'content-type': 'application/json', 'content-length': '188'}>
bytearray(b'[{"name":"0_transformworkflowtriton","version":"1","state":"READY"},{"name":"1_predictpytorchtriton","version":"1","state":"READY"},{"name":"executor_model","version":"1","state":"READY"}]')
[{'name': '0_transformworkflowtriton', 'version': '1', 'state': 'READY'},
{'name': '1_predictpytorchtriton', 'version': '1', 'state': 'READY'},
{'name': 'executor_model', 'version': '1', 'state': 'READY'}]
If all models are loaded successfully, you should be seeing READY
status next to each model.
from merlin.systems.triton.utils import send_triton_request
response = send_triton_request(workflow.input_schema, filtered_batch, model.output_schema.column_names)
print(response)
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Cell In[26], line 2
1 from merlin.systems.triton.utils import send_triton_request
----> 2 response = send_triton_request(workflow.input_schema, filtered_batch, model.output_schema.column_names)
3 print(response)
File /usr/local/lib/python3.8/dist-packages/merlin/systems/triton/utils.py:226, in send_triton_request(schema, inputs, outputs_list, client, endpoint, request_id, triton_model)
224 triton_inputs = triton.convert_table_to_triton_input(schema, inputs, grpcclient.InferInput)
225 else:
--> 226 triton_inputs = triton.convert_df_to_triton_input(schema, inputs, grpcclient.InferInput)
228 outputs = [grpcclient.InferRequestedOutput(col) for col in outputs_list]
230 response = client.infer(triton_model, triton_inputs, request_id=request_id, outputs=outputs)
File /usr/local/lib/python3.8/dist-packages/merlin/systems/triton/__init__.py:88, in convert_df_to_triton_input(schema, batch, input_class, dtype)
68 def convert_df_to_triton_input(schema, batch, input_class=grpcclient.InferInput, dtype="int32"):
69 """
70 Convert a dataframe to a set of Triton inputs
71
(...)
86 A list of Triton inputs of the requested input class
87 """
---> 88 df_dict = _convert_df_to_dict(schema, batch, dtype)
89 inputs = [
90 _convert_array_to_triton_input(col_name, col_values, input_class)
91 for col_name, col_values in df_dict.items()
92 ]
93 return inputs
File /usr/local/lib/python3.8/dist-packages/merlin/systems/triton/__init__.py:183, in _convert_df_to_dict(schema, batch, dtype)
181 else:
182 values = col.values if isinstance(col, pd.Series) else col.values_host
--> 183 values = values.reshape(*shape).astype(col_schema.dtype.to_numpy)
184 df_dict[col_name] = values
185 return df_dict
File /usr/local/lib/python3.8/dist-packages/pandas/core/arrays/masked.py:471, in BaseMaskedArray.astype(self, dtype, copy)
469 # to_numpy will also raise, but we get somewhat nicer exception messages here
470 if is_integer_dtype(dtype) and self._hasna:
--> 471 raise ValueError("cannot convert NA to integer")
472 if is_bool_dtype(dtype) and self._hasna:
473 # careful: astype_nansafe converts np.nan to True
474 raise ValueError("cannot convert float NaN to bool")
ValueError: cannot convert NA to integer
The response contains scores (logits) for each of the returned items and the returned it ids.
response.keys()
dict_keys(['item_id_scores', 'item_ids'])
Just as we requested by setting the top_k
parameter, only 20 predictions are returned.
response['item_ids'].shape
(15, 20)
This is the end of the tutorial. You successfully
performed feature engineering with NVTabular
trained transformer architecture based session-based recommendation models with Transformers4Rec
deployed a trained model to Triton Inference Server, sent request and got responses from the server.
References
Merlin Transformers4rec: https://github.com/NVIDIA-Merlin/Transformers4Rec
Merlin NVTabular: https://github.com/NVIDIA-Merlin/NVTabular/tree/stable/nvtabular
Merlin Dataloader: https://github.com/NVIDIA-Merlin/dataloader
Triton inference server: https://github.com/triton-inference-server